
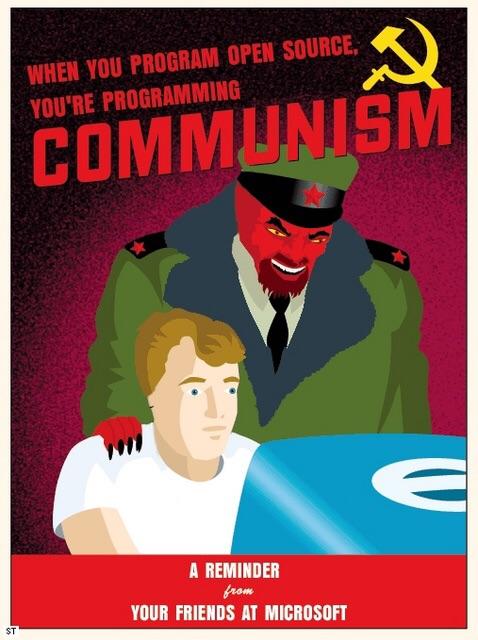
It’s still not entirely done (missing Cubemaps) and it’s only a part of a larger project. I’ve been working on this project off and on for the past 4-ish years.
spoiler
I’m heavily using SIMD to rasterize triangles and all the buffers (minus the frame buffer) are swizzled in a Z pattern for better cache hits. Shadow mapping is rather noisy in some cases. It also supports normal maps and specular reflections.
(Excuse the text having a fully transparent background)
This reads like it was written by some LLM.
Don’t ever disable journaling if you value your data.
Neither of these schedulers exist anymore unless you’re running a really ancient Kernel. The “modern” equivalents are
none
andbfq
. Also this doesn’t even touch on the many tunables thatbfq
brings.Also changing them like they suggest isn’t permanent. You’re supposed to set them via udev rules or some init script.
None of this changes any settings like they imply.
No shit. Who would’ve thought that throwing more/better hardware at stuff will make things faster.